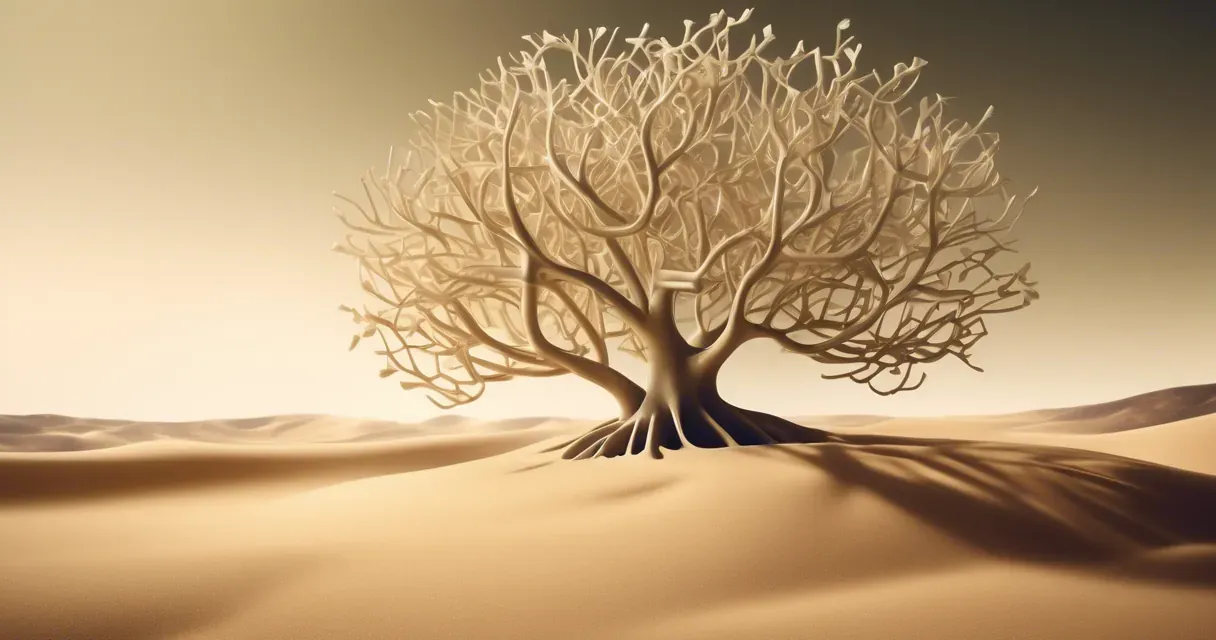
Secure Hierarchical Key Derivation System Implemented in Rust
/ 3 min read
Quick take - The article discusses the implementation of a secure hierarchical key derivation system in Rust, highlighting essential concepts, techniques for deriving master and child keys, the importance of secure coding practices, and the necessity of thorough testing to safeguard sensitive data.
Fast Facts
- Key Concepts: Familiarity with Extended Secret Key, hardened vs. non-hardened derivation, PBKDF2, and zeroization is essential for secure key derivation in Rust.
- Master Key Derivation: The master key is derived from a seed using PBKDF2, establishing a secure foundation for generating child keys.
- Child Key Derivation: Hardened derivation enhances security by using the parent’s secret key, while non-hardened derivation uses the parent’s public key, preventing exposure of private keys.
- Testing and Validation: Thorough testing is crucial to ensure accurate derivation of master and child keys, helping to identify potential vulnerabilities.
- Recommended Tools: The Rust programming language is favored for its performance and memory safety, with the Cargo.toml file aiding in project configuration and dependency management.
Implementing Secure Hierarchical Key Derivation in Rust: A Comprehensive Guide
In the ever-evolving landscape of cryptographic security, implementing a secure hierarchical key derivation system is paramount for protecting sensitive data. Recent tutorials have shed light on the essential practices needed to develop such a system using the Rust programming language. These resources emphasize the importance of secure coding techniques and effective key management.
Understanding Core Concepts
Before embarking on implementation, developers must grasp several foundational concepts. Key among these are the Extended Secret Key, hardened vs. non-hardened derivation, PBKDF2, and zeroization. The Extended Secret Key struct plays a pivotal role by encapsulating both a secret key and a chain code. Zeroization, or the practice of clearing sensitive data from memory once it is no longer needed, is crucial to prevent potential leaks of private keys or other sensitive information.
Master Key Derivation Process
The process begins with deriving the master key from a seed using PBKDF2, which employs HMAC-SHA512 to generate a secure secret key and chain code. This step forms a robust foundation for subsequent key generation. Once the master key is established, child keys can be derived through two distinct methods: hardened and non-hardened derivation.
Child Key Derivation Techniques
The tutorial delineates between these two methods clearly. Hardened key derivation uses the parent’s secret key combined with an index, adding an extra layer of security against potential attacks. Conversely, non-hardened derivation utilizes the parent’s public key, allowing for the derivation of child public keys without exposing private keys. This distinction is particularly significant for enhancing security in publicly accessible systems.
Developers are cautioned against common pitfalls during implementation. Neglecting zeroization can lead to unintentional information leaks. Furthermore, misunderstanding the differences between hardened and non-hardened derivation methods may result in exposing private keys, especially in sensitive contexts.
Testing and Validation
Thorough testing is indispensable to ensure the reliability of the key derivation process. The tutorial includes functions designed to validate that master keys, child keys, and public keys are accurately derived and verified. This testing phase is critical for identifying vulnerabilities or bugs that might otherwise remain undetected.
Tools and Resources
To aid in developing a secure hierarchical key derivation system, the tutorial recommends leveraging the Rust Programming Language. Known for its performance and memory safety features, Rust is particularly well-suited for cryptographic applications. The Cargo.toml file serves as a configuration hub for Rust projects, enabling developers to manage dependencies and ensure compatibility with cryptographic libraries.
By adhering to these guidelines and utilizing recommended tools, developers can significantly enhance the security of cryptographic wallets and safeguard sensitive data from potential threats.